Java Programming: Lesson 1: Variables
- Milpitas Xtreme Robotics
- Jul 31, 2018
- 6 min read
Updated: Aug 19, 2018
Welcome to programming! At the end of this course, you will be ready to start programming a competition ready FTC robot in Java. It is heavily recommended that you practice at home with fun exercises, as simply knowing the Java language/syntax doesn't necessarily mean you will know how to approach certain problems during your programming experience. That being said, let's dive into some code!
Data Types and Variables
A summary on variables and datatypes:
When programming, you will eventually need to hold data (such as numbers) to perform more complex tasks such as telling a robot how many degrees to move its wheels, or telling a servo which angle to stay in. These can be done through assigning data types held in variables. Just like in math class, variables are placeholders used to hold data for later use. The only difference is in programming, the data a variable holds can scale beyond simple numbers and mathematical equations! A variable can also hold words, letters, and even whole chunks of code (more into that later)! Since you are beginning to program, we will cover the three basic data types all programmers learn, regardless of what language they are learning to program in, and how they can be stored in variables for future use in your code. These three data types are: - Integers: Whole numbers ranging from -2,147,483,648 to 2,147,483,647
- Integers are usually used to perform mathematical calculations, store numerical data for further use, or even be used to count/track an amount of something. Integers are not only limited to mathematical computation but this is an introduction to what they can be used for, just to give you a general idea.
- Booleans: Either true or false
- Booleans are literally either true or false. They are used to execute conditional
code (lines/sections of code that only run on a certain condition ie: True or False).
These conditions could be used to tell a robot to move its arm up only if the A
button being pressed is true and the B button being pressed is false, or telling a
robot to move its arm down if the B button being pressed is true and the A being
pressed is false. Booleans don't neccesarily have to be only for tasks like these
but this is an introduction to what they can be used for, just to give you a general
idea.
- Strings: A series of character (including numbers) acting like "words"
- String are like words in programming. They represent a grouping of any character on your keyboard simply as those groups of characters and nothing else. If you had a variable that held Integer datatypes and you gave it a 5, it would be completely different from a variable that held String datatypes and was given a 5. This is because the Integer variable will have the 5 act as a number yet the String variable will have 5 act as a character. Strings aren't just limited to act as a group of characters but this will give you a general idea as to what they are and how they can be used.
Syntax for Variables and Datatypes:
Basic Syntax Declaration
Now that you know the basics of variable, data types, and how they can be used in code, you'll need to know how to write them in code so the computer understands what you are telling it to do. This may be intimidating at first but after a couple of practice exercises, you'll be a pro! To declare a variable, you will first need to indicate what types of values it will be used to hold: - For Integers, you will need to type: int.
- For Booleans, you will need to type: boolean.
- Finally, for Strings, you will need to type: String.
In all cases, indicating the datatype of a variable is case sensitive, so if you do not indicate the datatype of the variable you will be creating in the way I specified issues will arise in your code. And last but not least, variables have a following format for being created: (data type) (variable name) = (value) (;) - int number = 5;
- String name = "name";
- boolean bool = false; Examples:

Explanation: This example shows us how to create the basic data types we discussed, how to assign them values, and how to add comments!
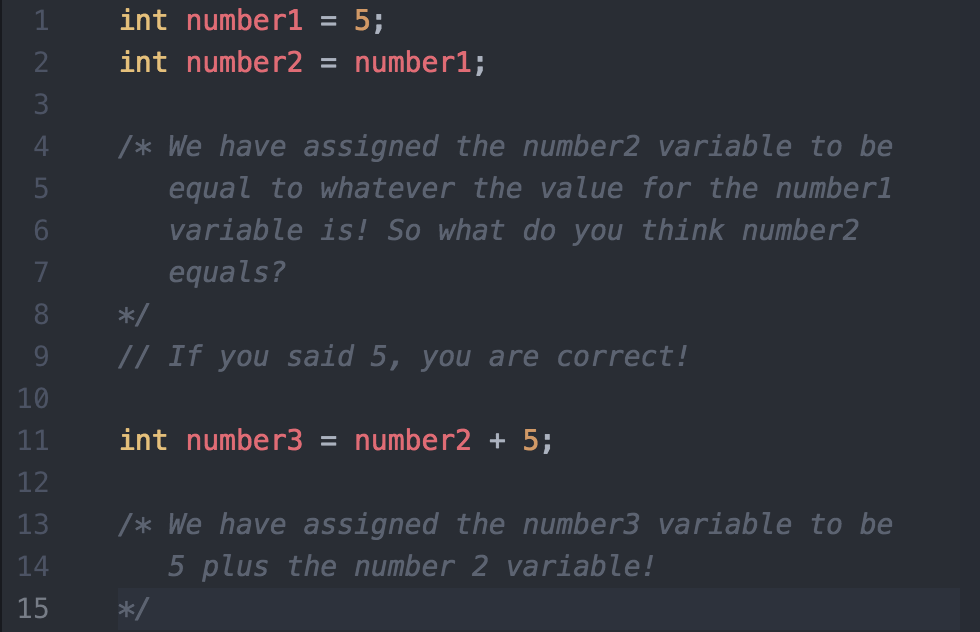
Explanation: This example shows us how variables can be equal to other variables, as long as their datatypes are the same! This also shows us how basic math operations such as +, -, /, * can be used in Java and even in variable creation!
Mid Lesson Challenge 1: 1. Create an Integer variable called number1 and give it the value 6
2. Create an Integer variable called number2 and give it the value of number1 plus 6 3. Create an Integer variable called number3 and give it the value 2 * 6
4. Create a String variable called name and give it your name as a value
5. Create a Boolean variable called value and assign it the value false Printing Values onto the Screen Now that you are comfortable with creating variables and giving them values, we will talk about how to print those values onto the screen in front of you. In technical terms, the screen used for printing and typing things onto the screen is known as the console, and the command to print anything to the console is as follows: - System.out.println( (what you want to print) ); - Whatever you want to print will be put in between those parenthesis
Examples:
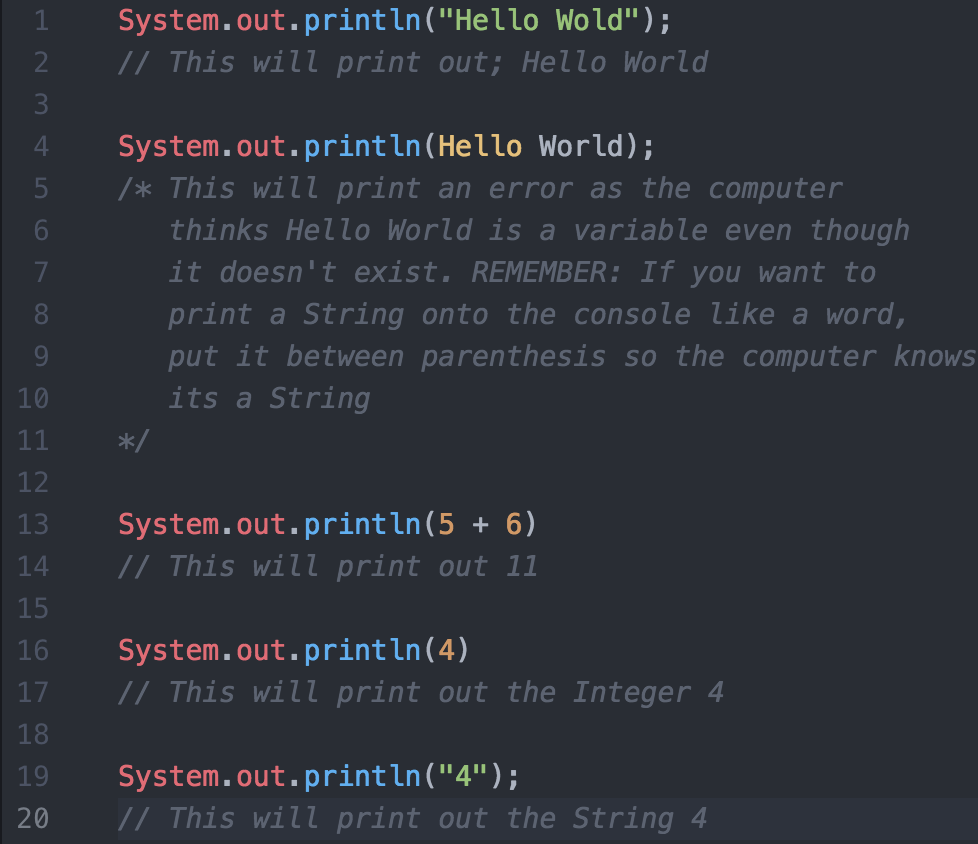
Explanation: This show different ways the System.out.println() command can be used such as with numbers, strings, math operations (which we will cover in the next section and lesson), and even variables (although not shown here). Also shows how the computer will perceive data types which may look the same
like 4 vs. "4" (4 is an Integer while "4" is a String)
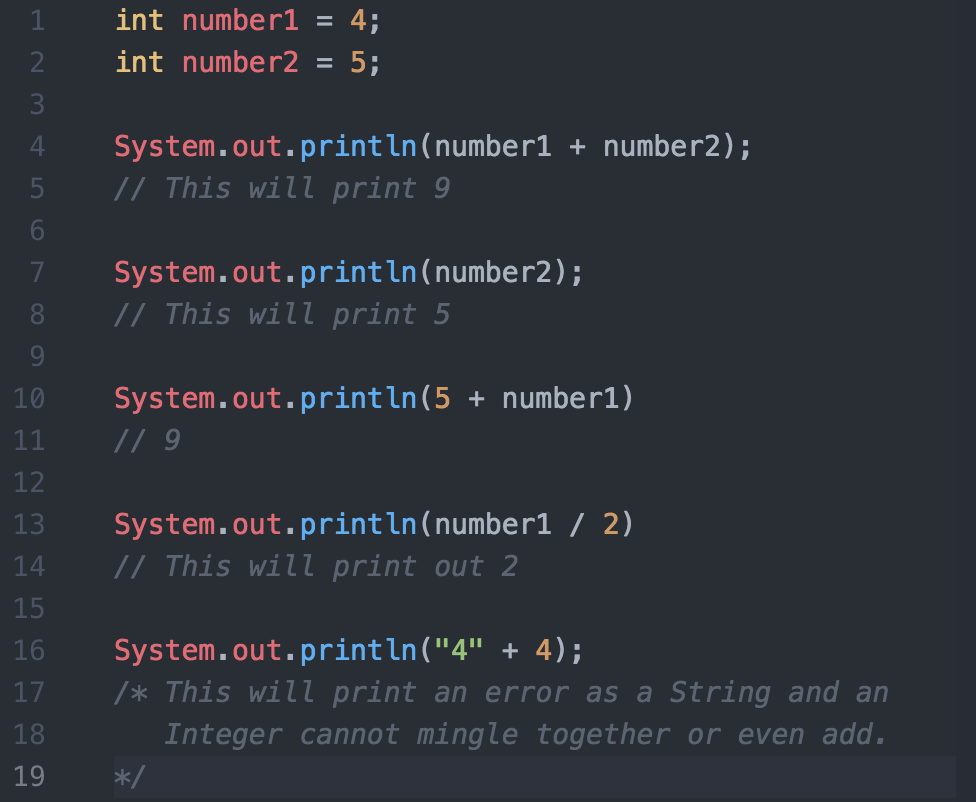
Explanation: This shows how the System.out.println() command can also print out variables and math operations between numbers and variables. It also outlines how different data types cannot interact such as adding a String and an Integer.
Mid Lesson Challenge 2:
1. Create two integer variables and name them whatever you want along with a value 2. Print the sum of those two values onto the screen 3. Print the string Hello World onto the screen
4. Create a boolean called value and give a true value
5. Print the variable value onto the screen
Intro to Basic Math Functions
As seen in previous sections of the lessons, Java has mathematical functions programmers can use to create equations, relationships between variables, and even run certain sections of software which demand mathematical computation/equations. For now, we will only focus on 5 major mathematical functions you will encounter alot when programming. - Addition: Addition is symbolized as two values being joined with a + sign - Subtraction: Subtraction is symbolized as two values being joined with a - sign - Multiplication: Multiplication is symbolized as two values being joined with a * sign - Division: Division is symbolized as two values being joined with a / sign
- Modulo: Modulo is unique in that it returns the remainder of division between two
numbers, symbolized as two number joined together by a % sign
Examples:
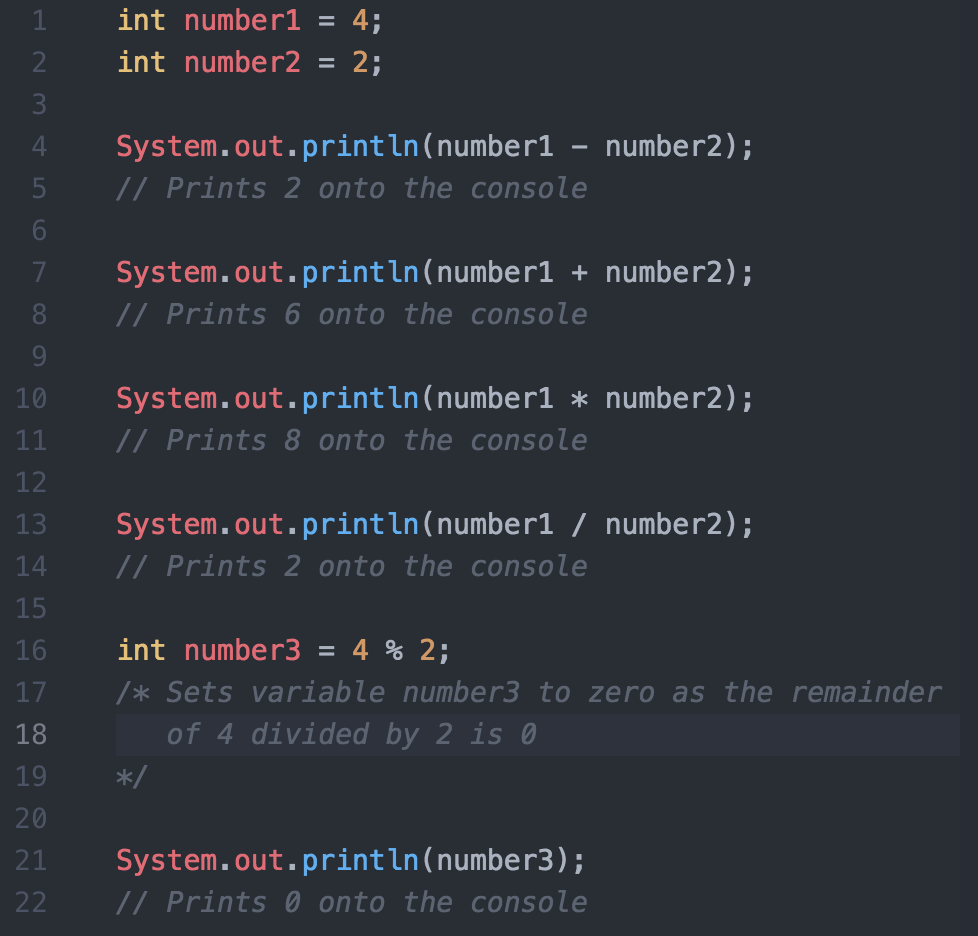
Explanation: This shows the different ways you can perform mathematical functions between either variables or even numbers! It also shows the outcome of each operation as expected and how to print them to the screen in different ways. (NOTE: Java follows PEMDAS so if you want to create equations with more than two numbers, you can! Here is a quick example:) int equation = (4 + 2) * 3;
Mid Lesson Challenge 3:
1. Create two integer variable that are divisible by each other
2. Print the sum of the two variables onto the console
3. Print the difference of the two variables on the console
4. Print the module between the two variables onto the console
5. Have the first variable become equal to itself times the second variable
6. Print the first variable onto the screen
Congrats! You've finished the first lesson for Java programming! You are one step closer to know how to program anything you want in Java like a pro. Remember, the only way you can get better is through practice, practice, and... practice :D! Find something you wanna make and see what skills you know you can use to solve that problem.
Problem Solutions: Challenge 1 Solution:
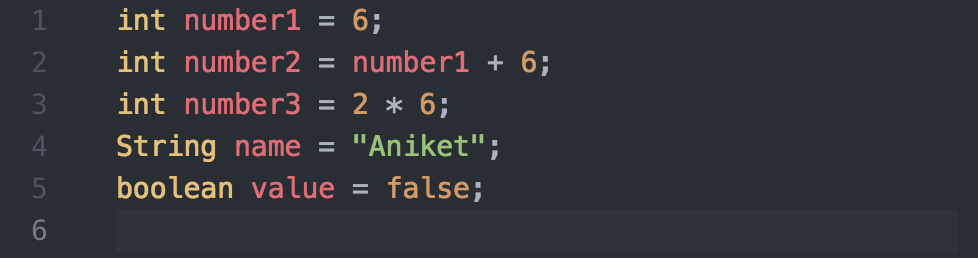
Explanation: This is the following solution to challenge 1 using the new syntax we learned in this lessons introduction.
Challenge 2 Solution:
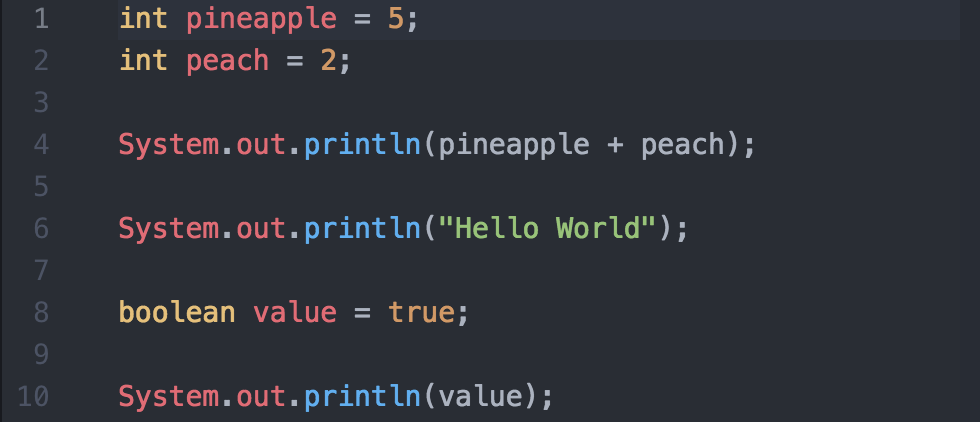
Explanation: This is the following solution to challenge 2 using our new knowledge of the System.out.println() command alongside some math functions.
Challenge 3 Solution:
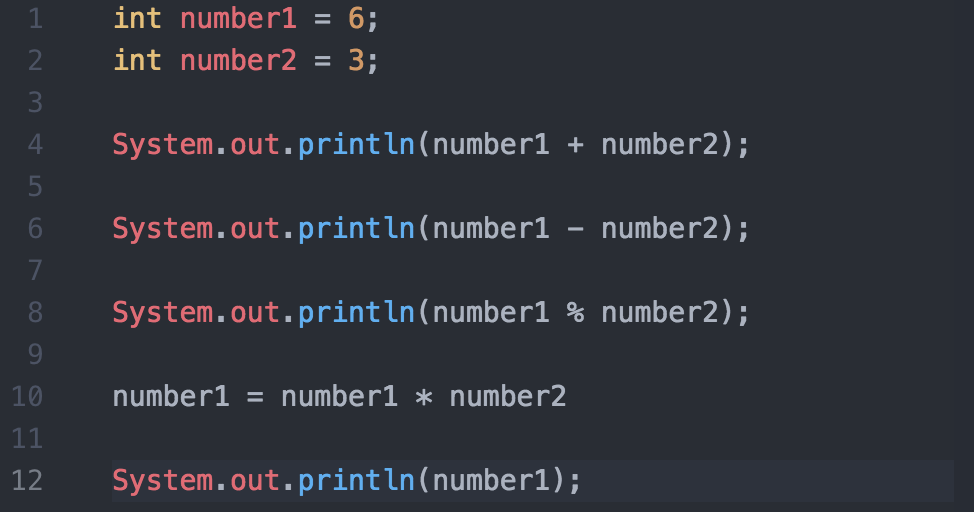
Explanation: This is the following solution to challenge 3 using our reviewed knowledge of math functions in Java and our now acquired knowledge of printing onto the console.
Congratulations! You have just finished the introductory lesson to Java programming! You will now be able:
- Create int, String, and boolean variables
- Assign variables different values and change their values mid-code
- Print values onto the console
- Utilize Math functions in:
- Console - Variables
- Pure numbers
- PEMDAS
Discuss what you thought about the lesson and how I can improve it! Also, if you have any questions, shoot them down in the comments or DM me on the MXR discord server or my discord account. Happy programming!